Architecture Diagram
Original Architecture:
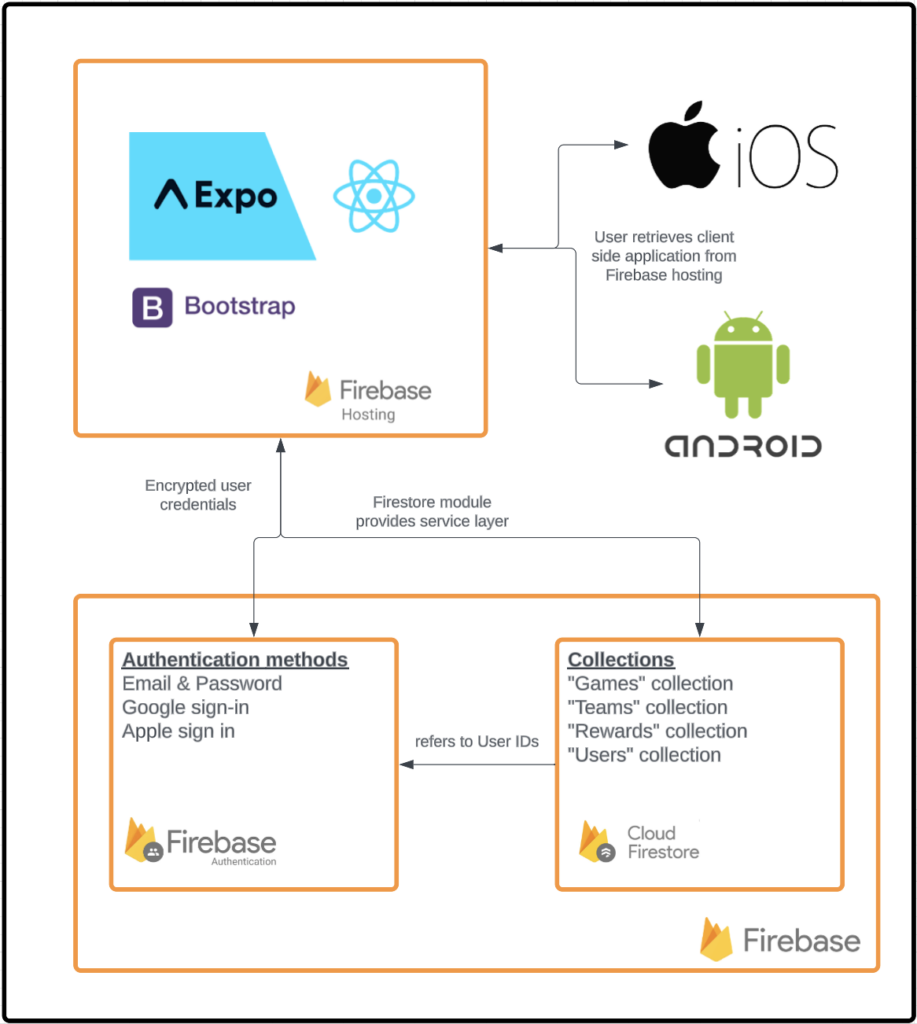
Final Architecture:
Code Repository
View our Github Repo!
View our README!
This project was made using TypeScript as the main programming language, React Native for the frontend technology, Firebase for our backend technology, Expo for our development environment, and VSCode as our IDE. Our final product combines the two apps created by the previous team for fans and admins.
View the annotated architecture diagram in the previous section for notes on the relationships between modules in our components!
Detailed Data Definitions
Collections:
- Game
- gameID: string
- The unique game ID
- gameState: string
- Describes the current state of the game. The possible states are: inactive, lobby, question, leaderboard, and finalLeaderboard
- currentQuestion: number
- The index of the current question
- questions: Question[]
- An array of resolved Question documents that can be used for that game
- startTime: Timestamp
- The starting time of the game
- team1: Team
- A resolved Team document representing the first team playing
- team2: Team
- A resolved Team document representing the second team playing
- team1score: number
- The total points accumulated by fans who chose team 1
- team2score: number
- The total points accumulated by fans who chose team 2
- team1responses: number
- The number of fans who played for team 1
- team2responses: number
- The number of fans who played for team 2
- gameID: string
- Question
- questionID: string (default empty string)
- The unique question ID
- answers: string[] (default [”])
- An array of the answer choices for this question
- points: number (default 0)
- The number of points the question is worth
- question: string (default empty string)
- The trivia question being asked
- questionTime: number (default 0)
- The amount of time given to answer the question
- correctAnswer: number (default 0)
- The index of the correct answer choice
- questionID: string (default empty string)
- Team
- teamID: DocumentReference (doc(FIRESTORE, ‘teams/’ + ‘defaultTeamId’)
- The unique team ID
- color1: string (default ‘gray’)
- The team’s main representative color
- color2: string (default ‘gray’)
- The team’s secondary representative color
- logo: string (default ‘default_logo_url’)
- A link for the team logo
- name: string (default ‘Unknown Team’)
- The team name
- teamID: DocumentReference (doc(FIRESTORE, ‘teams/’ + ‘defaultTeamId’)
- User
- email: string
- A valid email for the user
- firstName: string
- The user’s first name
- lastName: string
- The user’s last name
- role: string
- The user’s role
- userID: string
- The user’s unique ID
- username: string
- The user’s username
- email: string
Design Rationale: Design Decisions
We chose to use Firebase, Expo, React Native, and TypeScript to develop our app, which gave us the ability to quickly develop an app for iOS and Android devices. For a detailed description of our platform decision, see the Platform Selection page on our project website.
Firebase allowed us to create No-SQL document-based collections. This choice gives us more schema flexibility and the opportunity to scale horizontally as traffic increases on the app . Initially, we had Game, Question, Team, and User collections. As we developed, we decided to move the questions collection inside of the game collection as a subcollection. This design choice made it much simpler to create questions, since we could implement question creation as a part of game creation. It also makes it easier to remove questions in deleted games, since all unused questions will be deleted when the game is deleted. This choice also makes sense for the application of the FanzPlay app, since each game will have questions specific to that matchup that are not likely to be continuously reused.
We had a lot of trouble initially deciding how we would keep score for the game. At first, we thought we would need a new GamePlay collection that we would write to each time a user answered a question tracking the game, question, team the user was playing for, and whether their response was correct or incorrect. This would mean that we would need to query the GamePlay collection each time we calculated the team score and individual score, grouping by various fields. This would lead to Instead, we chose to keep track of individual scores locally, since individual scores are only shown to that user. To keep track of team score, we added fields in the Game collection to keep track of correct responses and total responses for each team. This saves us storage and computation costs when compared to using the GamePlay. It also allows us to quickly calculate and display team scores using just the Game document. We used Firebase increment functions to alter these fields, ensuring consistency, even in the presence of concurrent updates.
Another design choice we made was to use modals instead of routes for the game screens and admin update screens. Using modals made it easier to keep track of data and states, and it also gives users increased focus, as the modal fills the entire screen and hides the app navigation bar.
Check out some of our other deliverables: Specifications, User Manual, Test Plan