Installation:
- Required Installations prior to running app
- VS Code or other IDE
- Navigate to this link https://code.visualstudio.com/download for VScode installation. Click on one of the download links depending on what Operating System the user is on.
- NPM and NodeJS
- Navigate to https://nodejs.org/en/download and download the right installer for your operating system.
- React Native
- Navigate to https://reactnative.dev/docs/environment-setup and follow the environment set-up and getting started tutorials.
- VS Code or other IDE
Set up:
- Clone the Repository into Local development platform (for us, VSCode):
- After installation, navigate to the GitHub repository then, click on the green Code button followed by download ZIP.
- Extract the zip file using your favorite extractor. We recommend WinRAR or 7Zip.
- Open VSCode. Use keyboard shortcut Ctrl + Shift + P (Windows) or Command + Shift + P (Mac) to open the Command Palette bar at the top.
- Type “Git: Clone” into the Command Palette bar to pull up the option, then paste the URL into the bar.
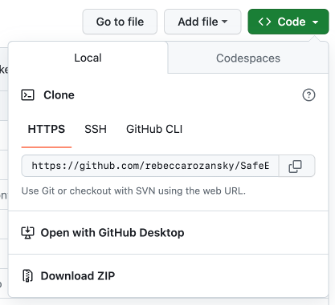
- Setting up your environment:
- Launch VS Code.
- In the top bar, go to File > Open Folder, then find the folder that was produced from the extraction earlier.
- In the top bar, go to Terminal > New Terminal. A terminal should appear at the bottom part of the screen as shown.
- In the terminal, type in “npm install” without the quotes. This might take a while.
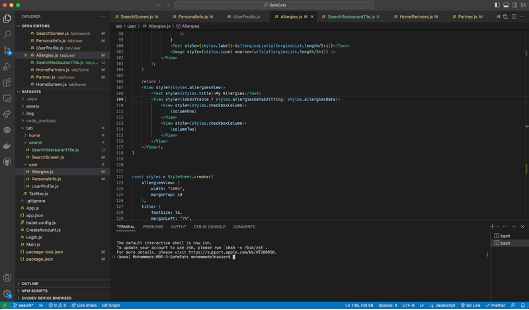
- Launching Prerequisites:
- iOS Simulator:
- You will need a MacOS device in order to run it for iOS.
- Go to the Apple Store and download xCode.
- Launch xCode, then go to the top bar and click on Xcode > Preferences.
- A new screen will pop up. Click on Components and then download one of the simulators.
- Android and iOS devices:
- Download Expo Go on the Google Play Store or Apple Store
- iOS Simulator:
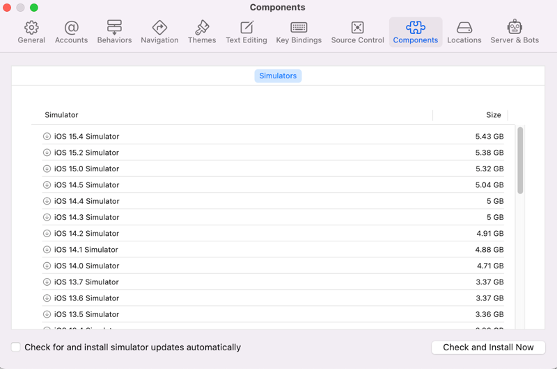
- Launching the app:
- First, create a file under the ‘config’ folder called firebase.js and copy and paste the following code snippet into the file
// Import the functions you need from the SDKs you need
import { initializeApp } from "firebase/app";
import { getAnalytics } from "firebase/analytics";
import { getAuth } from "firebase/auth";
import { getDatabase } from 'firebase/database';
// TODO: Add SDKs for Firebase products that you want to use
// https://firebase.google.com/docs/web/setup#available-libraries
// Your web app's Firebase configuration
// For Firebase JS SDK v7.20.0 and later, measurementId is optional
const firebaseConfig = {
apiKey: "AIzaSyBmS4jgjGrVqWPB42U49KCQefk4XPZXNVw",
authDomain: "safeeats-95af3.firebaseapp.com",
projectId: "safeeats-95af3",
storageBucket: "safeeats-95af3.appspot.com",
messagingSenderId: "810418533490",
appId: "1:810418533490:web:31c42d61014b906434feeb",
measurementId: "G-G0ZSFZEZMD",
databaseURL: "https://safeeats-95af3-default-rtdb.firebaseio.com/"
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
const auth = getAuth(app);
const analytics = getAnalytics(app);
const db = getDatabase(app);
export { app, auth, db};
- Your screen should now look like this
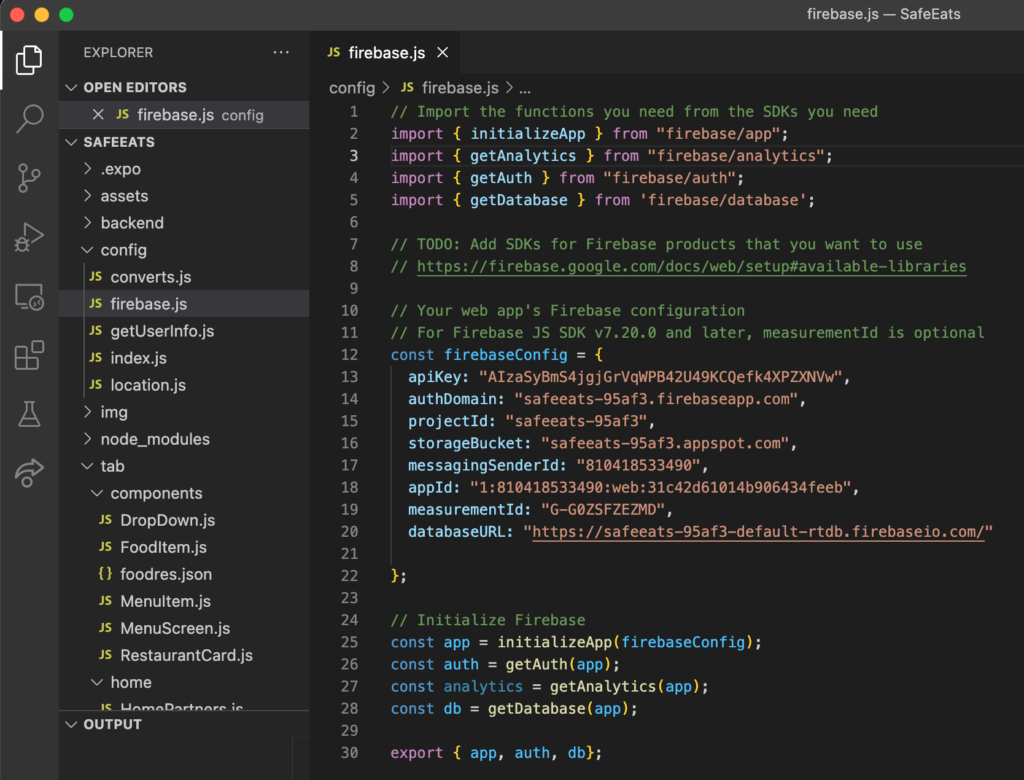
- Go to the terminal and type “npm run start”
- From there you can scan the QR code to run the app on your phone.
- You can also press i to run the app on the iOS simulator
Technical Descriptions:
The SafeEats App was developed with the React-Native JavaScript framework. We chose React Native because it’s open source, has great documentation, and has great compatibility with other applications. To store the menu data, we use Firebase which offers powerful, simple, and cost-effective object storage. They work together to provide users with the ability to login, search menus near the user, and store profile preferences. Github was used to store and manage code.
- React-Native offers the ability to create native apps for iOS and Android platforms with a single codebase, resulting in reduced development time and cost.
- Firebase is a platform developed by Google that provides various services and tools for building and managing mobile and web applications. It offers features such as backend services, cloud storage, real-time database, authentication, hosting, analytics, and more.
- VSCode is a code editor that lets us edit, manage, and update our code in a clean and simple manner. Many different code editors exist, and each has their perks. If you’re familiar with other code editors, feel free to use them. Just know that our initial directions are written for VSCode users.
- Git is an open-source library that lets us do version control on our code and share our code with each other. GitHub is a web-based, code hosting platform that enables users to collectively edit and manage code together.